In JavaScript, a ‘Multi-dimensional’ or ‘Nested’ array is created when sub-arrays are placed within an outer array. JavaScript lacks a specific format for creating nested arrays, so sub-arrays are nested within a single outer array. The elements of inner arrays are accessed through their index in the outer array.
Once you’ve declared a nested array in JavaScript, you can perform various operations on it. These operations include appending sub-arrays, accessing elements within sub-arrays, iterating through sub-array elements, deleting sub-arrays or their related elements, and reducing the dimensionality of the nested array.
In this article, we’ll explore how nested arrays work in JavaScript, with the help of practical examples to illustrate their functionality. Let’s get started!
How to Create a Nested Array in JavaScript?
Here’s how to create a nested array in JavaScript:
const nestedArray = [[inner_array1], [inner_array2], [inner_array3]];
JavaScriptIn the given example, “array” signifies the outer array, which contains multiple inner arrays referred to as “inner_array1,” “inner_array2,” and “inner_array3.
Example: Creating a Nested Array in JavaScript
In this example, we’ll create a multi-dimensional or nested array named “hobbies.” This “hobbies” array will include five inner arrays, each representing different sets of hobbies or interests.
let hobbies = [
['Reading', 4],
['Gardening', 2],
['Gaming', 1],
['Painting', 8],
['Cooking', 5]
];
JavaScriptIn the declared ‘hobbies‘ array, the first dimension represents the ‘hobby,’ and the second dimension indicates the maximum number of ‘hours’ spent while doing that activity.
To display the created ‘hobbies‘ nested array, we will use the ‘console.table()’ method, passing the ‘hobbies’ array as an argument:
console.table('hobbies' );
JavaScriptRunning the code provided above will display the contents of the ‘hobbies’ array in a table format. The first column will represent the index of inner arrays, while the other two columns will contain the elements located at the first ‘[0]‘ and second ‘[1]‘ indexes, respectively.

How to access elements of nested arrays in JavaScript?
Looking to access elements within a nested array in JavaScript? If so, check out the following syntax:
Array.[a][b]
JavaScriptIn this context, ‘a’ corresponds to the index of the ‘inner’ array within the created nested array, while ‘b’ denotes the index of the ‘element’ within the specified inner or sub-array.
Example: Accessing Elements in Nested Arrays with JavaScript
Let’s consider a scenario where we aim to access the “Cooking” hobby, which is the “First” element at index [0] within the fifth inner array at index [4].
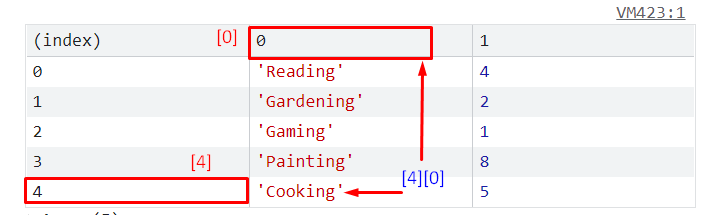
To carry out this particular operation, you can execute the following code statement:
console.log('hobbies' [4][0]);
JavaScriptFrom the displayed output, it’s evident that we’ve successfully accessed the value of the ‘hobbies’ array, which is located at the first index of the fifth inner array.
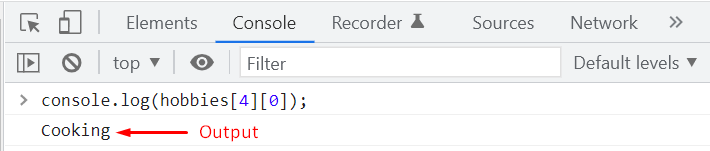
How to add elements to nested array in JavaScript?
In JavaScript, there are two methods to add elements to an existing nested array. You can either append an element at the end of the array using the ‘push()’ method or insert it at a specific position using the ‘splice()’ method.
Example: Adding Elements to a Nested Array in JavaScript.
To append the ‘[Cycling, 6]’ sub-array at the end of the ‘hobbies’ nested array, you can achieve this by passing it as an argument to the ‘hobbies.push()’ method:
hobbies.push(['Cycling', 6]);
console.table(hobbies);
JavaScriptWhen the ‘hobbies.push()’ statement is executed, it will add the specified sub-array at the end of the ‘hobbies’ array.
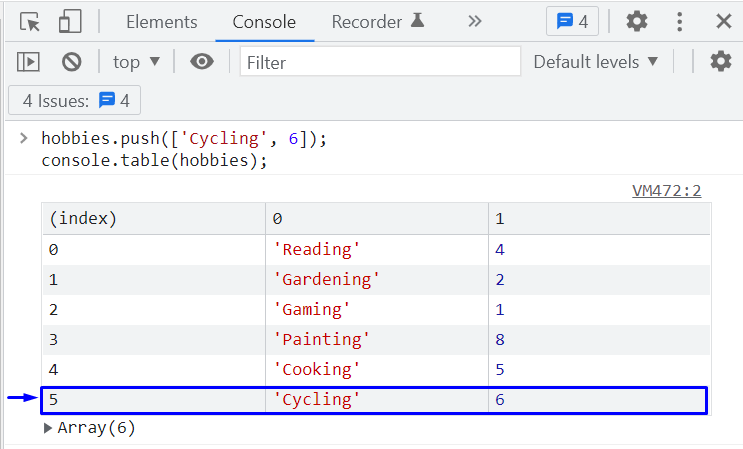
To insert a sub-array in the middle of other inner arrays, you can use the ‘splice()’ method in the following manner:
hobbies.splice(1, 0, ['Singing', 3]);
console.table(hobbies);
JavaScriptHere, the ‘hobbies.splice()’ method will modify the ‘hobbies’ array, inserting the ‘[‘Singing’, 3]’ sub-array at the second position.
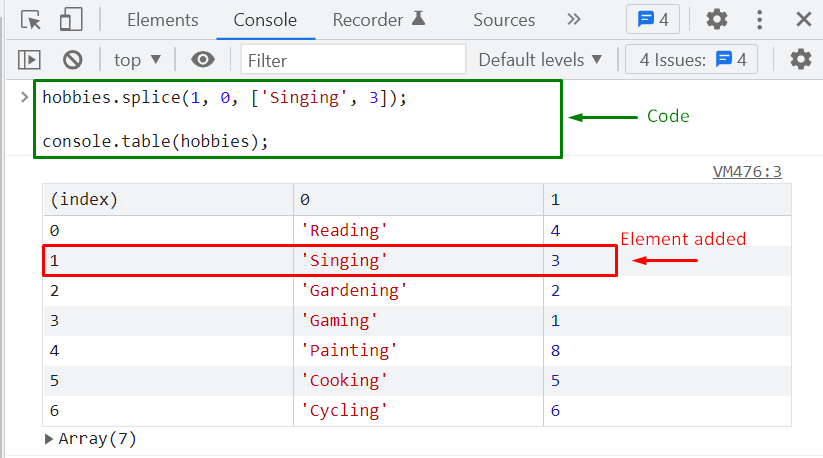
Up to this point, we’ve covered the process of creating a nested array and adding elements to it. In the next section, we’ll delve into iterating over the elements of a nested array in JavaScript.
How to iterate over the elements of nested array in JavaScript?
While the JavaScript ‘for’ loop is commonly used to iterate over the elements of an array, in the case of a ‘nested’ array like ours, we’ll employ two ‘for’ loops nested within each other for effective iteration.
Example: Iterating Over the Elements of a Nested Array in JavaScript
The outer ‘for’ loop iterates over the elements of the outer array based on its size, while the nested ‘for’ loop performs the iteration over the inner sub-arrays:
/**
* Iterate through a 2D array of hobbies and log each element's indices and value.
* @param {Array<Array<any>>} hobbies - The 2D array of hobbies to iterate through.
*/
for (let i = 0; i < hobbies.length; i++) {
/**
* Length of the inner array at index i.
* @type {number}
*/
var innerArrayLength = hobbies[i].length;
for (let j = 0; j < innerArrayLength; j++) {
/**
* Log the indices and value of each element in the 2D array.
* @type {string}
*/
console.log('[' + i + ',' + j + '] = ' + hobbies[i][j]);
}
}
JavaScriptThe provided iteration operation will output all elements of the ‘hobbies’ nested array.
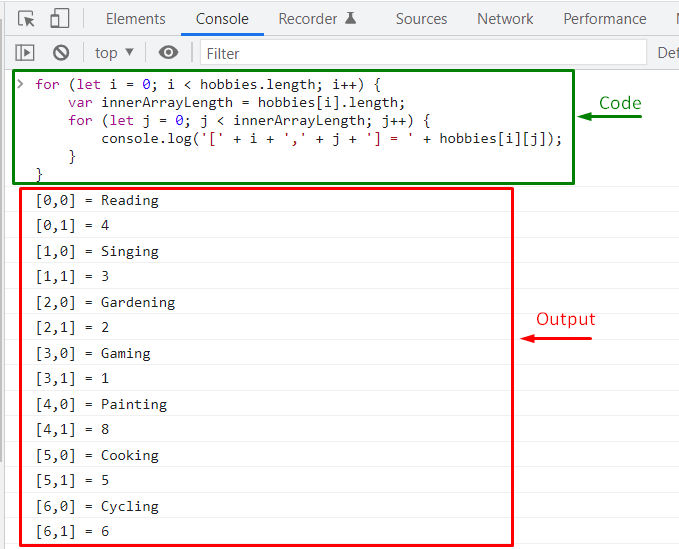
How to flatten a nested array in JavaScript
In certain scenarios, you might encounter the need to create an array that encompasses all elements of a nested JavaScript array in their original order. In such cases, flattening the created nested array is essential to reduce its dimensionality.
The Array.flat() method, introduced in ES6, serves as a built-in solution for flattening a nested JavaScript Array. This method returns a new array obtained by concatenating all the elements of the sub-arrays.
Example: Flattening a Nested Array in JavaScript
For example, to flatten the ‘hobbies’ array, you can execute the following code in the console window:
const flatArray= hobbies.flat();
console.log(flatArray);
JavaScriptThe hobbies.flat() method, when applied, will reduce the dimensionality of the ‘hobbies’ array, effectively flattening the elements of the inner arrays.
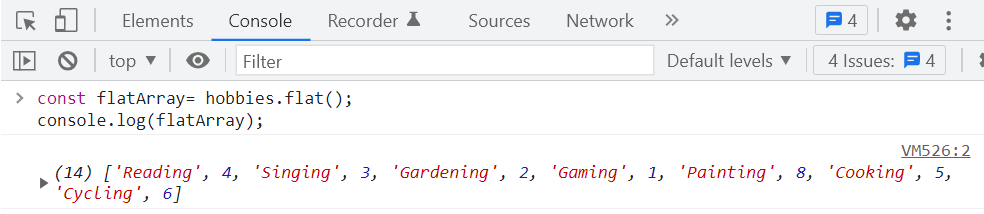
How to delete elements of nested array in JavaScript?
To remove elements from any sub-arrays of a nested array, you can utilize the ‘pop()’ method. The ‘pop()’ method is commonly used to delete the last inner-array from a nested array, but it is also effective for removing elements from inner arrays.
Example: Deleting Elements from a Nested Array in JavaScript
Before employing the ‘pop()’ method, the ‘hobbies’ nested array contains the following sub-arrays.
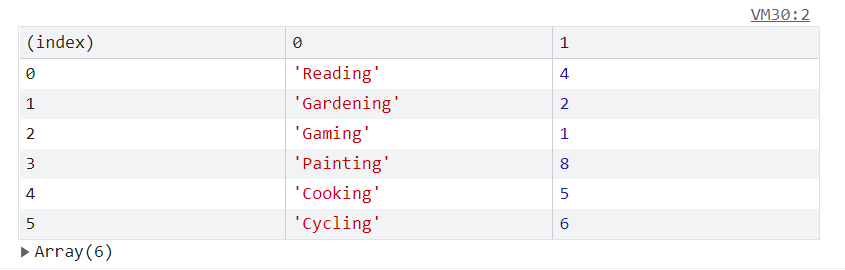
Now, upon invoking the ‘pop()’ method, the last sub-array will be deleted along with its elements:
hobbies.pop();
console.table(hobbies);
JavaScript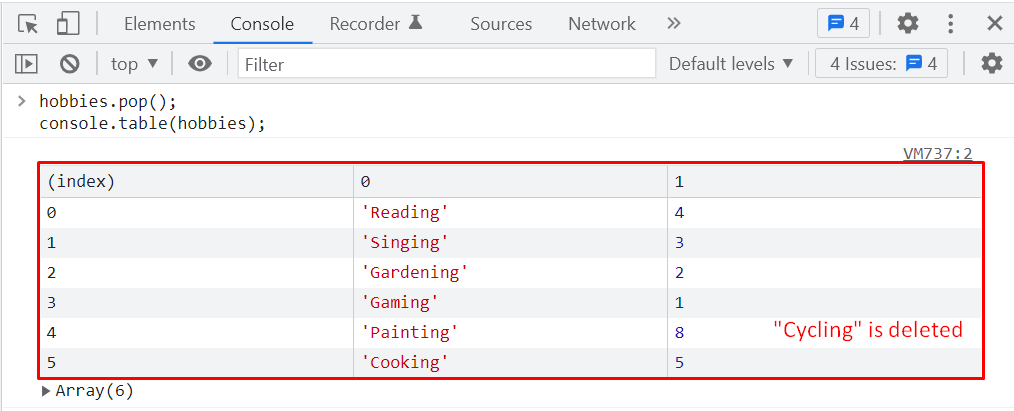
To remove the second element of each sub-array, we can iterate through the ‘hobbies’ array using the ‘forEach()’ method. During each iteration, the ‘pop()’ method can be employed to delete the element located at the first index:
/**
* Remove the last element from each inner array in the hobbies array.
* @param {Array<Array<any>>} hobbies - The 2D array of hobbies to modify.
*/
hobbies.forEach((hobby) => {
hobby.pop(1);
});
// Move console.table() outside the forEach loop to display the modified array
console.table(hobbies);
JavaScriptAs observed in the provided output, the element representing the maximum number of hours spent on each hobby has been deleted for all sub-arrays.
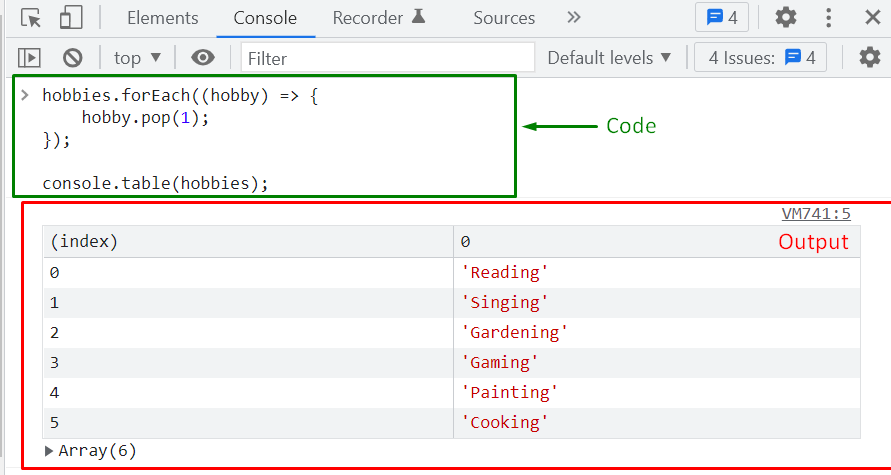
We have covered all the essential information related to the functioning of nested arrays in JavaScript. Feel free to explore and experiment with them based on your preferences.
Conclusion
In JavaScript, the term “nested array” refers to the inclusion of an inner array or sub-array within an outer array. After creating a nested array, essential operations include using the “push()” and “splice()” methods for adding elements, employing “for loops” and “forEach()” methods to iterate over inner array elements, applying “flat()” method to reduce dimensionality, and utilizing “pop()” method to delete sub-arrays or their elements. This write-up provided an overview of working with nested arrays in JavaScript.